Here's an example of how you could create a multicolor loading circle using ImGui in C++:
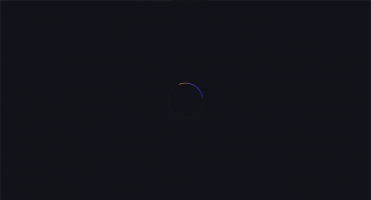
This code creates a new ImGui window and draws a loading circle with 12 segments, each with a different hue value (based on the current time). The circle is drawn using the ImDrawList interface, which provides a low-level API for drawing shapes and text in ImGui. The AddTriangleFilled function is used to draw each segment of the loading circle, while the AddTriangle function is used to draw the outline of each segment. The GetColorU32 function is used to generate an ImU32 color value from an ImVec4 color vector, which is needed by the AddTriangleFilled and AddTriangle functions.
The loading circle is centered in the middle of the ImGui window and has a radius of 20 pixels. The thickness of the circle segments is set to 4 pixels. You can customize these values to suit your needs.
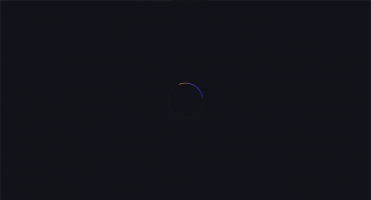
C++:
#include <imgui.h>
void MyImGuiFunction()
{
// Start a new ImGui window
ImGui::Begin("My Window");
// Set up the loading circle parameters
const float circleRadius = 20.0f;
const float circleThickness = 4.0f;
const ImVec2 circleCenter = ImGui::GetCursorScreenPos() + ImVec2(circleRadius, circleRadius);
// Draw the loading circle
const int numSegments = 12;
const float segmentAngle = 2.0f * 3.1415926f / numSegments;
const float t = ImGui::GetTime();
for (int i = 0; i < numSegments; i++)
{
const float segmentStartAngle = i * segmentAngle;
const float segmentEndAngle = (i + 0.5f) * segmentAngle;
const ImVec2 segmentStart(cosf(segmentStartAngle), sinf(segmentStartAngle));
const ImVec2 segmentEnd(cosf(segmentEndAngle), sinf(segmentEndAngle));
const ImU32 color = ImGui::GetColorU32(ImVec4::HSV(i / (float)numSegments, 0.8f, 0.8f));
ImDrawList* drawList = ImGui::GetWindowDrawList();
drawList->AddTriangleFilled(
circleCenter + segmentStart * circleRadius,
circleCenter + segmentEnd * circleRadius,
circleCenter,
color
);
drawList->AddTriangle(
circleCenter + segmentStart * circleRadius,
circleCenter + segmentEnd * circleRadius,
circleCenter,
ImGui::GetColorU32(ImGuiCol_Border),
circleThickness
);
}
// End the ImGui window
ImGui::End();
}
This code creates a new ImGui window and draws a loading circle with 12 segments, each with a different hue value (based on the current time). The circle is drawn using the ImDrawList interface, which provides a low-level API for drawing shapes and text in ImGui. The AddTriangleFilled function is used to draw each segment of the loading circle, while the AddTriangle function is used to draw the outline of each segment. The GetColorU32 function is used to generate an ImU32 color value from an ImVec4 color vector, which is needed by the AddTriangleFilled and AddTriangle functions.
The loading circle is centered in the middle of the ImGui window and has a radius of 20 pixels. The thickness of the circle segments is set to 4 pixels. You can customize these values to suit your needs.
Last edited by a moderator: